project
A DOS® Server In a Virtual Machine
|
Making The Echo Protocol On Debian In PHP
In my own words, I want to retell the article about sockets in PHP as applied to Debian which is originally at http://i-novice.net/sokety-v-php/.
PHP is realized as a command interpreter on Debian. *.php files should be executable. In general, they can be run from a command-line interface (CLI) as follows,
php file.php
We're going to make the Echo Protocol which is a service in the Internet Protocol Suite defined in RFC 862. We shall need two modules: a server ("server.php") and a client ("client.php"). Either of them runs on one and the same machine.
Do as follows,
mkdir ~/client-server; touch ~/client-server/server.php; chmod +x ~/client-server/server.php; touch ~/client-server/client.php; chmod +x ~/client-server/client.php
Then, do the following:
nano ~/client-server/server.php
Copy and paste the following code in "server.php":
<?php
header('Content-Type: text/plain;');
error_reporting(E_ALL ^ E_WARNING);
set_time_limit(0);
ob_implicit_flush();
echo "-= Server =-\n\n";
$address = 'localhost';
$port = 10001;
try {
echo 'Create socket ... ';
if (($sock = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) < 0) {
throw new Exception('socket_create() failed: '.socket_strerror(socket_last_error())."\n");
} else {
echo "OK\n";
}
echo 'Bind socket ... ';
if (($ret = socket_bind($sock, $address, $port)) < 0) {
throw new Exception('socket_bind() failed: '.socket_strerror(socket_last_error())."\n");
} else {
echo "OK\n";
}
echo 'Listen socket ... ';
if (($ret = socket_listen($sock, 5)) < 0) {
throw new Exception('socket_listen() failed: '.socket_strerror(socket_last_error())."\n");
} else {
echo "OK\n";
}
do {
echo 'Accept socket ... ';
if (($msgsock = socket_accept($sock)) < 0) {
throw new Exception('socket_accept() failed: '.socket_strerror(socket_last_error())."\n");
} else {
echo "OK\n";
}
$msg = "Hello, Client!";
echo "Say to client ($msg) ... ";
socket_write($msgsock, $msg, strlen($msg));
echo "OK\n";
do {
echo 'Client said: ';
if (false === ($buf = socket_read($msgsock, 1024))) {
throw new Exception('socket_read() failed: '.socket_strerror(socket_last_error())."\n");
} else {
echo $buf."\n";
}
if (!$buf = trim($buf)) {
continue;
}
if ($buf == 'shutdown') {
socket_close($msgsock);
break 2;
}
echo "Say to client ($buf) ... ";
socket_write($msgsock, $buf, strlen($buf));
echo "OK\n";
} while (true);
} while (true);
} catch (Exception $e) {
echo "\nError: ".$e->getMessage();
}
if (isset($sock)) {
echo 'Close socket ... ';
socket_close($sock);
echo "OK\n";
}
?>
Save it.
Then:
nano ~/client-server/client.php
Copy and paste the following code in "client.php":
<?php
header('Content-Type: text/plain;');
error_reporting(E_ALL ^ E_WARNING);
set_time_limit(0);
ob_implicit_flush();
echo "-= Client =-\n\n";
$address = 'localhost';
$port = 10001;
try {
echo 'Create socket ... ';
$socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP);
if ($socket < 0) {
throw new Exception('socket_create() failed: '.socket_strerror(socket_last_error())."\n");
} else {
echo "OK\n";
}
echo 'Connect socket ... ';
$result = socket_connect($socket, $address, $port);
if ($result === false) {
throw new Exception('socket_connect() failed: '.socket_strerror(socket_last_error())."\n");
} else {
echo "OK\n";
}
echo 'Server said: ';
$out = socket_read($socket, 1024);
echo $out."\n";
$msg = "Hello, Server!";
echo "Say to server ($msg) ...";
socket_write($socket, $msg, strlen($msg));
echo "OK\n";
echo 'Server said: ';
$out = socket_read($socket, 1024);
echo $out."\n";
$msg = 'shutdown';
echo "Say to server ($msg) ... ";
socket_write($socket, $msg, strlen($msg));
echo "OK\n";
} catch (Exception $e) {
echo "\nError: ".$e->getMessage();
}
if (isset($socket)) {
echo 'Close socket ... ';
socket_close($socket);
echo "OK\n";
}
?>
Save it.
On Debian, open two SSH sessions: for the client and for the server.
In the server's, type as follows:
php ~/client-server/server.php
In the client's, do:
php ~/client-server/client.php
You'll see the following picture:
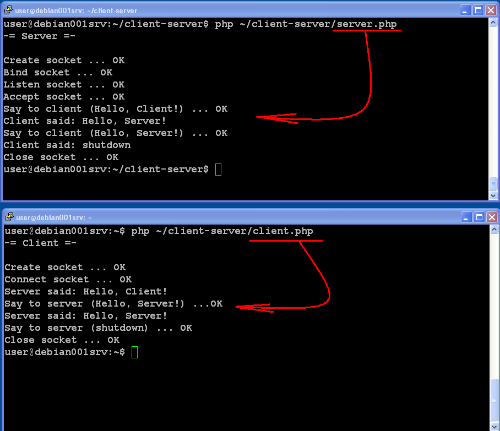
The Echo Protocol is working. Enjoy!
|
|